September 8, 2023
Best Practices and Tips for Structuring React.js Projects
Learn expert strategies to structure React.js projects effectively for better maintainability and scalability. Get tips tailored to project sizes with code examples.
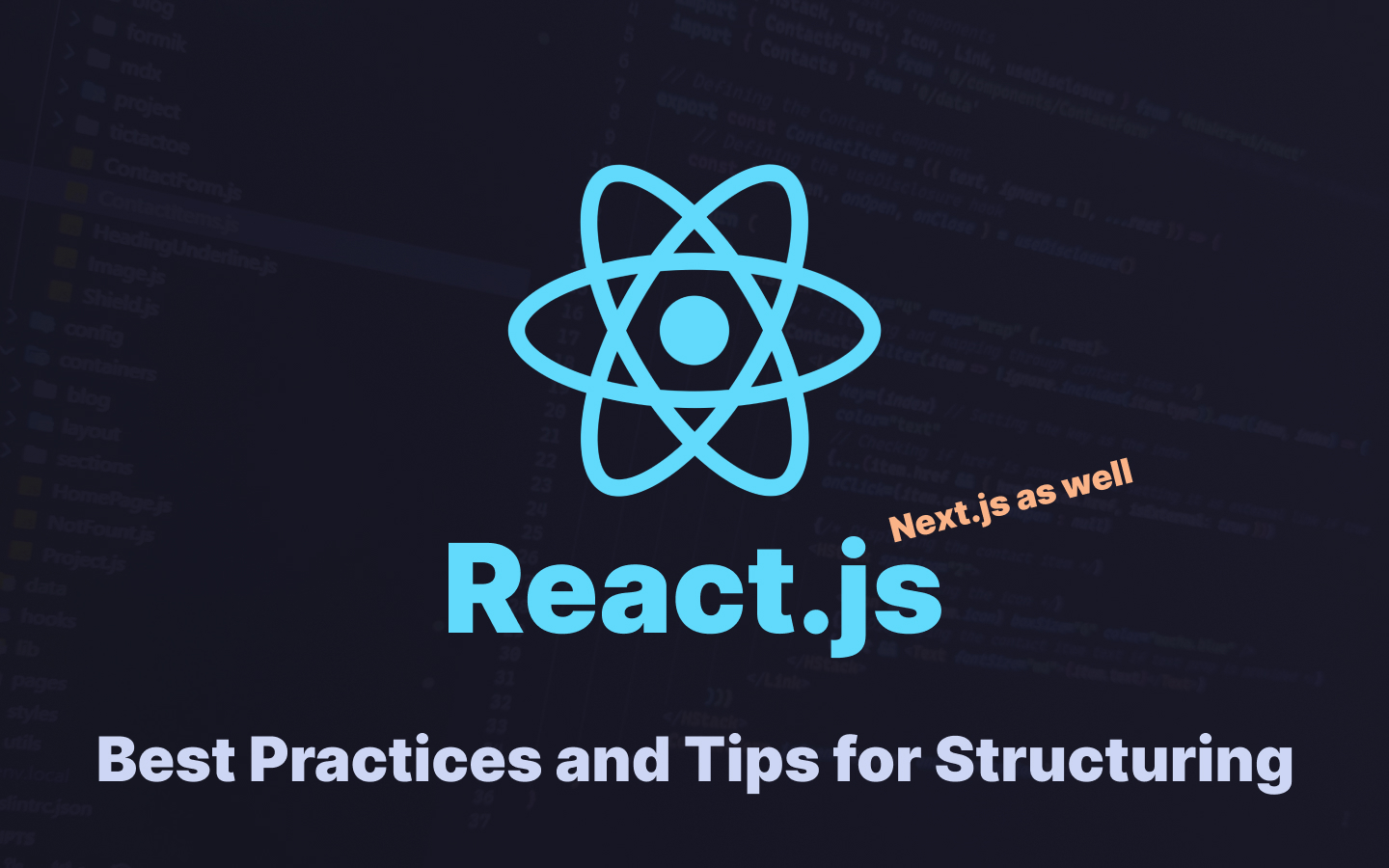
When it comes to building web applications with React.js, the way you structure your project can significantly impact its maintainability and scalability. In this blog post, we will delve into best practices and tips for structuring React.js projects, tailored to different project sizes – smaller, mid-size, and large-scale. To provide clarity, we'll accompany our recommendations with code blocks that illustrate the differences.
Note: This article applies to Next.js projects as well, since Next.js is built on top of React.js and uses a similar folder structure, the only difference is that _app.js in Next.js is App.js in React.js and there added _document.js files in Next.js.
Table of Contents
Smaller Projects
1. Folder Structure
In smaller React.js projects, simplicity is key. Consider the following folder structure:
src/
├── components/
├── pages/
├── utils/
├── App.js
└── index.js
- components: Store your reusable UI components here.
- pages: Define your main application pages.
- utils: Place utility functions and helper modules here.
- App.js: The main application entry point.
- index.js: The entry point of your application.
2. Component Organization
Keep your components organized by functionality. For example, if you have a "Header" component, place it in the components
folder. Use self-contained components that are easy to understand and maintain.
3. State Management
For smaller projects, you can manage state using React's built-in state or useState
hook. Avoid overcomplicating things with external state management libraries like Redux.
Mid-Size Projects
1. Folder Structure
As your project grows, consider a more modular structure:
src/
├── components/
│ ├── Button/
│ ├── Header/
│ └── ...
├── containers/
├── pages/
├── services/
├── utils/
├── App.js
└── index.js
- components: Split components into folders by feature.
- containers: Place container components here for managing data and interactions.
- services: Add API services and external integrations here.
2. State Management
For mid-size projects, consider using a state management library like Redux or Mobx. Centralize your application state for better scalability and data management.
3. Code Splitting
Leverage code splitting to load only the necessary code for each route, improving performance. React Router offers easy integration with code splitting.
Large-Scale Projects
1. Folder Structure
In large-scale React.js projects, a more organized structure is essential for maintainability:
src/
├── assets/
├── components/
│ ├── Button/
│ ├── Header/
│ └── ...
├── containers/
├── layouts/
├── pages/
│ ├── Home/
│ ├── Dashboard/
│ └── ...
├── services/
├── state/
├── utils/
├── App.js
└── index.js
- assets: Store static assets like images and fonts here.
- layouts: Define layouts for different sections of your app.
- state: Use a modular approach to state management. Each module handles its own state, actions, and reducers.
2. Routing
Implement a robust routing system using React Router to manage complex navigation in large apps. Create nested routes for better organization.
3. Testing and Documentation
Invest in thorough testing and documentation to ensure code quality and make it easier for your team to collaborate on a large-scale project.
Conclusion
By adhering to these best practices and tips, you can efficiently structure your React.js projects, ensuring they remain maintainable and scalable as they grow. Tailor your project structure to its size and complexity, and always aim for clean, organized code that promotes collaboration among your development team.