August 20, 2023
Understanding REST API Methods
Explore the five primary REST API methods: GET, POST, PUT, PATCH, and DELETE. Learn their purposes, usage, and best practices for designing and interacting with RESTful APIs.
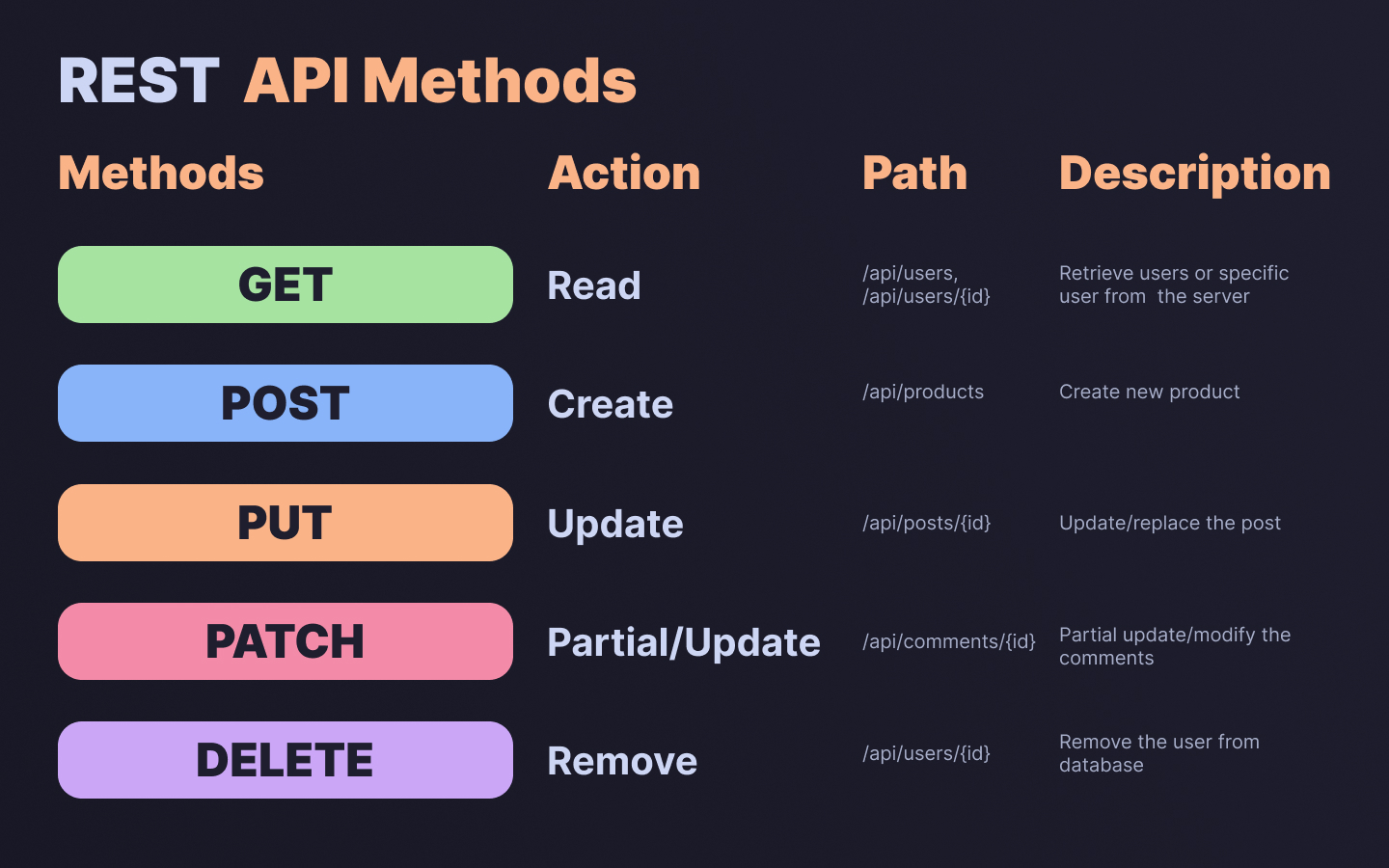
REST (Representational State Transfer) is a widely used architectural style for designing networked applications. One of its key features is the use of various HTTP methods to perform different actions on resources. In this blog post, we'll delve into the five primary REST API methods: GET, POST, PUT, PATCH, and DELETE.
Table of Contents
GET Method
The GET method is used to retrieve data from a server. It's a safe and idempotent operation, meaning that repeated calls to the same endpoint with the same parameters will produce the same result. This method does not modify any data on the server.
GET /api/users
--- OR ---
GET /api/users/123
POST Method
The POST method is used to submit data to be processed to a specified resource. It's often used to create new resources on the server. The data to be sent is included in the request body.
POST /api/products
Content-Type: application/json
{
"name": "New Product",
"price": 19.99
}
PUT Method
The PUT method is used to update or replace an existing resource with new data. It requires sending the complete updated data to the server, even if only a small part of the resource needs to be changed.
PUT /api/posts/123
Content-Type: application/json
{
"title": "Updated Title",
"content": "Updated content..."
}
PATCH Method
The PATCH method is used to apply partial modifications to a resource. Unlike PUT, which requires sending the complete updated data, PATCH allows you to send only the specific fields that need to be updated.
PATCH /api/comments/456
Content-Type: application/json
{
"content": "Updated comment content..."
}
DELETE Method
The DELETE method is used to request the removal of a resource from the server. It performs the deletion of the specified resource.
DELETE /api/users/789
Conclusion
Understanding the various REST API methods is essential for designing and interacting with RESTful APIs effectively. Each method serves a specific purpose and plays a role in creating, reading, updating, and deleting resources on a server.
Remember to use these methods appropriately and consider the idempotency and safety properties associated with each method to ensure predictable and reliable interactions with your API.